Authorization
To use pdf4me, you need to authenticate with basic authentication. You can get a free trial Api Key from our web portal. When using the Pdf4meApi directly you can set the basic authentication in the Header as following:
- Authentication: Basic <Your Key>
Please login with your social account or create an account here. Once logged in you have access to your free credentials. Authorization happens through the Pdf4meClient. The credential hand-over is language dependent.
Check the Subscription tab after logging in. Select the ‘Default’ application or create an application using ‘Create Application’.
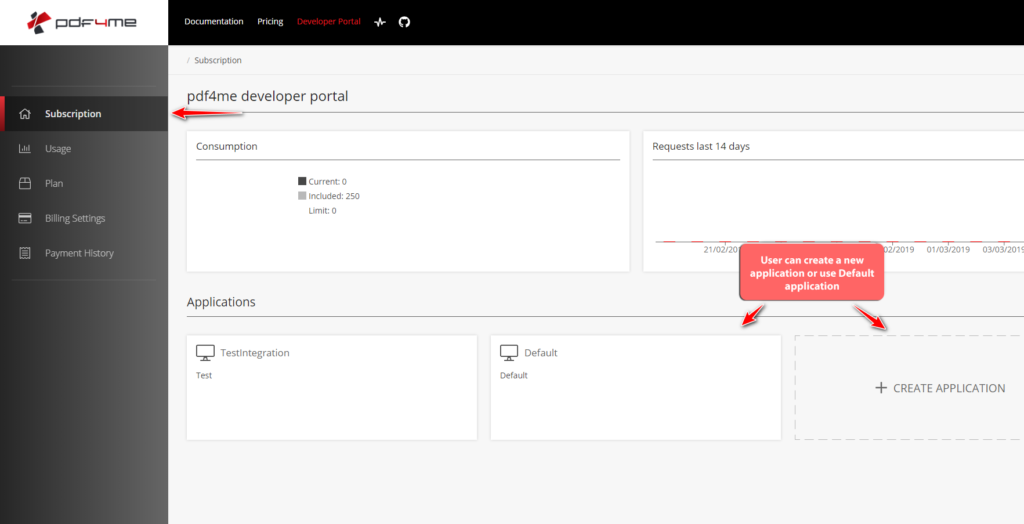
Copy the Primary/Scondary key and pass it to the authentication code (token).
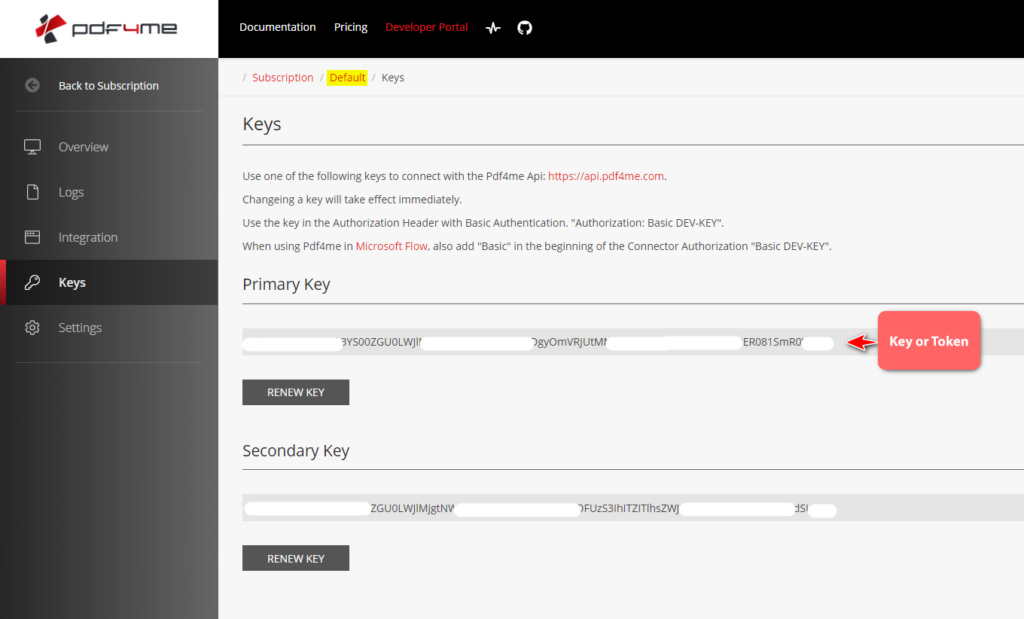
Sample
- curl
- C#
- Java
- JavaScript
- PHP
- Python
- Ruby
curl No Sample
using Pdf4meClient;
// You can provide a generated token to initialise authorization.
Pdf4meClient.Pdf4me.Instance.Init(token);
// The above setting need to be done only once.
// Rest of the client will be authenticated when accessing
// them through 'Pdf4me.Instance' object as shown below
byte[] file1 = File.ReadAllBytes("myFirstPdf.pdf");
byte[] file2 = File.ReadAllBytes("mySecondPdf.pdf");
byte[] mergedPdf = Pdf4me.Instance.MergeClient.Merge2PdfsAsync(file1, file2);
// writing it to disk
File.WriteAllBytes("mergedPdf.pdf", mergedPdf);
/*
Either you store them in the config.properties file with key token
Do not use any quotes:
Correct: token=sample-not-working-key-aaaaaaa
Incorrect:token="sample-not-working-key-aaaaaaa"
*/
import java.io.File;
import org.apache.commons.io.FileUtils;
import com.pdf4me.client.Pdf4meClient;
import com.pdf4me.client.MergeClient;
Pdf4meClient pdf4meClient = new Pdf4meClient();
/*
In case the location of your config.properties differs from the default
location, the source folder, provide the optional argument pathToConfigFile.
*/
Pdf4meClient pdf4meClient = new Pdf4meClient("resources/config.properties");
// or you pass them as arguments when constructing the Pdf4meClient object
Pdf4meClient pdf4meClient = new Pdf4meClient("https://api.pdf4me.com", token);
/*
The pdf4meClient object delivers the necessary authentication when instantiating
the different pdf4meClients such as for instance Merge.
*/
MergeClient mergeClient = new MergeClient(pdf4meClient);
byte[] mergedPdf = mergeClient.merge2Pdfs(new File("myFirstPdf.pdf"), new File("mySecondPdf.pdf"));
FileUtils.writeByteArrayToFile(new File("mergedPdf.pdf"), mergedPdf);
const fs = require('fs')
const pdf4me = require('pdf4me')
// replace 'YOUR API KEY' with your token
const pdf4meClient = pdf4me.createClient('YOUR API KEY')
const mergedPdf = await pdf4meClient.merge2pdfs(
fs.createReadStream('myFirstPdf.pdf'),
fs.createReadStream('mySecondPdf.pdf')
)
// writing it to disk
fs.writeFileSync('mergedPdf.pdf', mergedPdf)
// load Composer
require 'vendor/autoload.php';
use Pdf4me\API\HttpClient as pdf4meAPI;
$token = "6fg******jdS"; // replace this with your token
$client = new pdf4meAPI($token);
// The pdf4meClient object delivers the necessary authentication when instantiating the different pdf4meClients such as for instance Merge
$pdfMerge = $client->pdf4me()->merge2Pdfs([
"file1" => 'pdf2.pdf',
"file2" => 'pdf2.pdf'
]);
//writing it to file
file_put_contents('mergedPdf.pdf', $mergedPdf);
from pdf4me.client.pdf4me_client import Pdf4meClient
from pdf4me.client.merge_client import MergeClient
"""
Either you store them in the config.properties file with key token
Do not use any quotes:
Correct: token=sample-not-working-key-aaaaaaa
Incorrect: token="sample-not-working-key-aaaaaaa"
In case the location of your config.properties differs from the default location ('../config.properties'), provide the optional argument path_to_config_file.
"""
pdf4me_client = Pdf4meClient(path_to_config_file='path_to_my_config.properties')
""" or you pass them as arguments when constructing the Pdf4meClient object """
pdf4me_client = Pdf4meClient(token=token)
# The pdf4meClient object delivers the necessary authentication when instantiating the different pdf4meClients such as for instance Merge
merge_client = MergeClient(pdf4me_client)
merged_pdf = merge_client.merge_2_pdfs(
file1=open('my_first_pdf.pdf', 'rb'),
file2=open('my_second_pdf.pdf', 'rb')
)
//writing it to file
with open('merged.pdf', 'wb') as f:
f.write(merged_pdf)
# Pdf4me works with any Rack application or plain old ruby script. In any regular script the configuration of Pdf4me looks like
# - Require pdf4me
# - Configure pdf4me with API endpoints and credentials
# - Call appropriate methods
# - Require pdf4me
require 'pdf4me'
# - Configure pdf4me with API endpoints and credentials
Pdf4me.configure do |config|
# config.debugging = true
config.token = 'your-app-token'
end
# - Call appropriate methods, for instance merge 2 documents.
a = Pdf4me::MergeTwoPdfs.new(
file1: '/path/to/file/1.pdf',
file2: '/path/to/file/2.pdf',
save_path: 'merged.pdf'
)
a.run # safe returns true|false